Spring Boot – ResponseStatusException
reason not returned in response body
At some point I have decided that I really have to return HTTP codes for some illegal invocations of my API. I have decided to use simple solution.
@GetMapping("/responsestatusexception") public String getResourceThatThrowsResponseStatusException() { throw new ResponseStatusException( HttpStatus.NOT_FOUND, "Not found thrown by getResourceThatThrowsResponseStatusException"); }
however, this solution has one, small drawback. You have to remember about putting
server.error.include-message=always
inside application.properties. Otherwise, reason will not be passed back. You can read some thoughts about that here.
Anyways, before I have learned that I can use this approach, I have already built something different. Solution based on @ControllerAdvice. Here, I have a custom exception handler that extracts info from the exception and passes it back to client as JSON message.
@ControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(NotFoundException.class) public ResponseEntity<ErrorDTO> generateNotFoundException(NotFoundException ex) { ErrorDTO errorDTO = new ErrorDTO(); errorDTO.setMessage(ex.getMessage()); errorDTO.setStatus(String.valueOf(ex.getStatus().value())); errorDTO.setTime(new Date().toString()); return new ResponseEntity<ErrorDTO>(errorDTO, ex.getStatus()); } }
You can find full solution here:
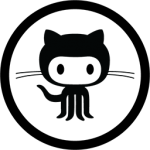